Our platform features a tagging system that assigns each problem to the companies that frequently ask it in interviews. Additionally, problems are organized by difficulty level, so you can gradually progress to more complex challenges. This means that you can focus your preparation on the specific types of problems that are most likely to be asked in your interviews, while also building up your skills and confidence with each new challenge. Whether you’re just starting out or you’re a seasoned pro looking to fine-tune your skills, our platform is designed to help you prepare effectively and efficiently. Our guides are also enriched with knowledge that has been carefully extracted from hundreds of hours of tutorials and guides available on other platforms.
The course is updated constantly and new videos/explanation every day which makes it a great pay-once keep-forever investment. Our approach is try it-watch it-read it-practice again-refresh-retain which enables you to go through problems explanations and videos, refresh your knowledge in 1-2minute and retain the information so you can apply it during the real interview. If you’re wondering if there are explanations using your favorite programming language, you should know that we currently have solutions for all problems in 4 most populater interview programming languages Java, C++, Python and Javascript.
What is the process that your team followed to create the list of interview questions on your platform?
Good question, here is the breakdown:
Become the System Design Interview King: The Only Guide You’ll Ever Need
- Industry-standard system design books, such as “Designing Data-Intensive Applications” by Martin Kleppmann and “System Design Interview” by Alex Xu
- Articles and blog posts by experienced system designers, such as the ones published by High Scalability and the Netflix TechBlog
- Real-world examples of system designs from popular tech companies, such as Uber, Amazon, and Google
- Most frequent questions collected from interview experience posts from multiple platforms
- 17 problems solved each broken into common patterns/topics you will learn in the fundamentals
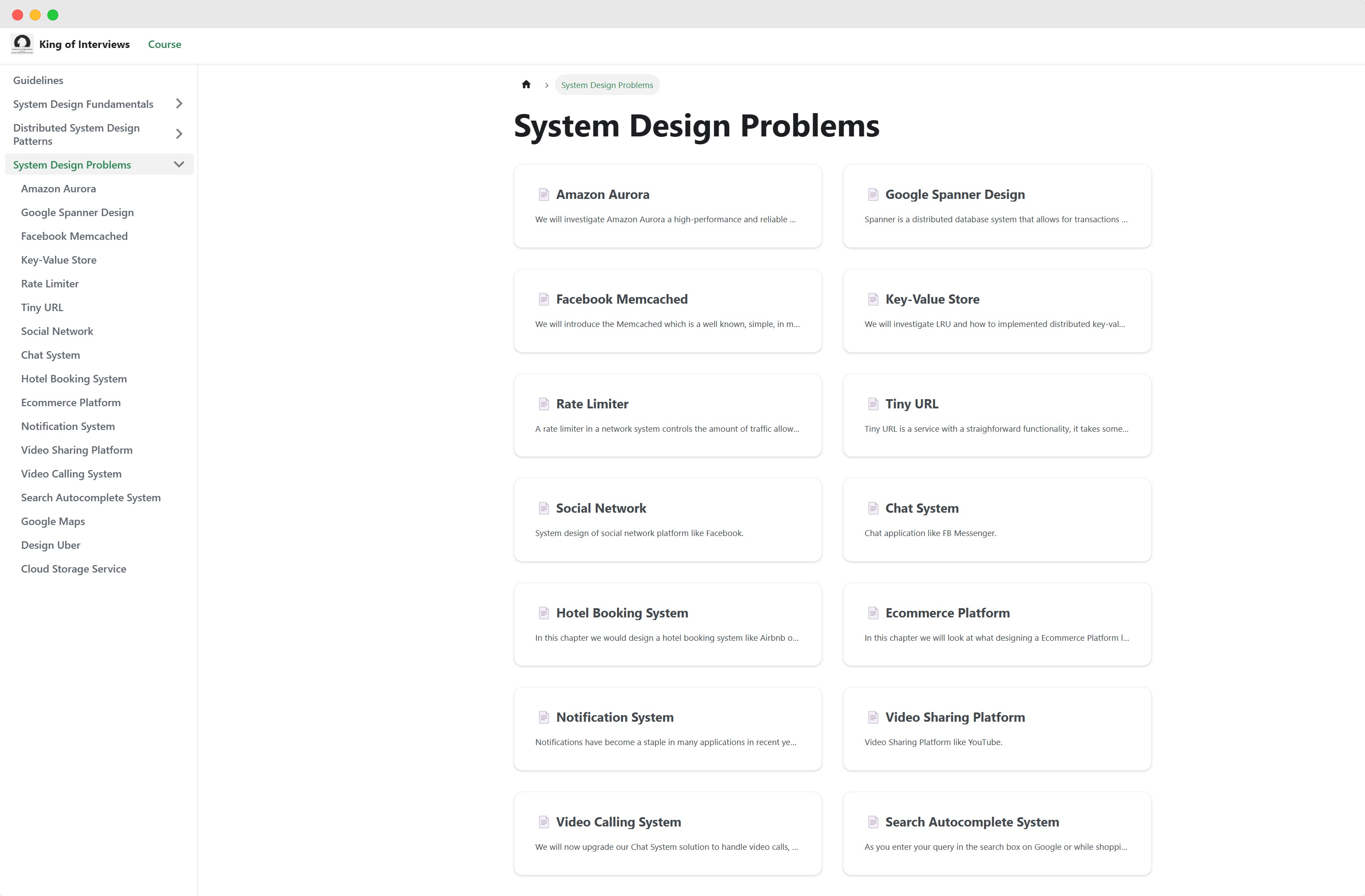
System Design Fundamentals
- Three Concerns in Sofware Systems
- Data Models and Query Language
- Storage and Retrieval
- Encoding and Evolution
- Replication
- Partitioning
- Transactions
- The Trouble with Distributed Systems
- Consistency and Consensus
- Batch Processing
- Stream Processing
- The Future of Data Systems
Distributed System Design Patterns
- Asynchronous Messaging
- AutoScalling
- Caching
- Data Consistency
- Data Partitioning
- Data Replication
- Telemetry
System Design Problems
- Amazon Aurora
- Google Spanner Design
- Facebook Memcached
- Key-Value Store
- Rate Limiter
- Tiny URL
- Social Network
- Chat System
- Hotel Booking System
- Ecommerce Platform
- Notification System
- Video Sharing Platform
- Video Calling System
- Search Autocomplete System
- Google Maps
- Design Uber
- Cloud Storage Service
- Classic computer science textbooks, such as “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein, and “The Algorithm Design Manual” by Steven S. Skiena
- Popular interview preparation books, such as “Cracking the Coding Interview” by Gayle Laakmann McDowell and “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- Online resources, such as LeetCode, HackerRank, and GeeksforGeeks
- Cross matched popular lists (like
Grind 75
) for common set of questions
- 200+ problems split into 15 categories available for free on
Algorithms and Data Structures Platform
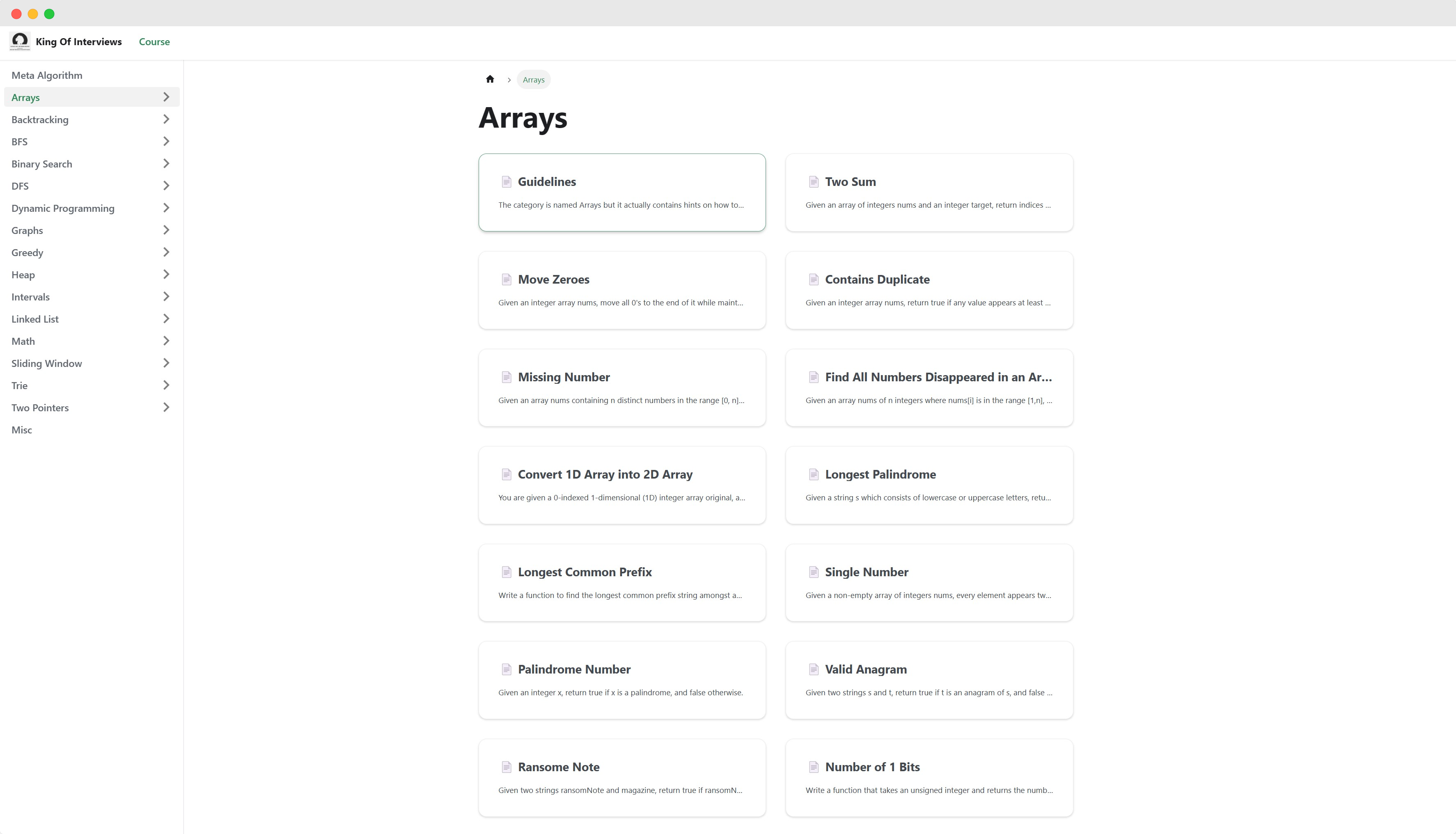
Meta Algorithm
Called Meta since it’s an algorithm defining how to use other algorithms (no shit Sherlock). But no seriolsy it’s like a memory palace to quickly respond to any problem and match problem to pattern you already know. Course is divided into categories that represent common patterns found in technical interviews, with guidelines on how to use them effectively.
Arrays
- Guidelines
- Two Sum
- Move Zeroes
- Contains Duplicate
- Missing Number
- Find All Numbers Disappeared in an Array
- Convert 1D Array into 2D Array
- Longest Palindrome
- Longest Common Prefix
- Single Number
- Palindrome Number
- Valid Anagram
- Ransome Note
- Number of 1 Bits
- Reverse Bits
- Add Binary
- Roman to Integer
- Majority Element
- Range Query Sum Immutable
- Valid Parentheses
- Implement Queue using Stacks
- Find All Duplicates In Array
- Find the Duplicate Number
- Min Stack
- String to Integer (atoi)
- Evaluate Reverse Polish Notation
- Set Matrix Zeroes
- Rotate Array
- Spiral Matrix
- Rotate Image
- Group Anagrams
- Product of Array Except Self
- Valid Sudoku
- Subarray Sum Equals K
- Contiguous Array
- Longest Consecutive Sequence
- Asteroid Collision
- Accounts Merge
- Daily Temperatures
- Largest Rectangle in Histogram
- First Missing Positive
Backtracking
- Guidelines
- Letter Case Permutation
- Letter Combinations of a Phone Number
- Subsets
- Subsets II
- Permutations
- Permutations II
- Combinations
- Combination Sum
- Combination Sum II
- Combination Sum III
- Word Search
- Palindrome Partitioning
- Genarate Parentheses
- Sudoku Solver
- N-Queens
BFS
- Guidelines
- Average of Levels in Binary Tree
- Minimum Depth of Binary Tree
- Binary Tree Level Order Traversal
- Binary Tree Level Order Traversal II
- Binary Tree Zigzag Level Order Traversal
- Binary Tree Right Side View
- Populating Next Right Pointers in Each Node
- Populating Next Right Pointers in Each Node II
- 01 Matrix
- Rotting Oranges
- Maximum Width of Binary Tree
- Word Ladder
Binary Search
- Guidelines
- Binary Search
- Find Smallest Letter Greater Than Target
- First Bad Version
- Find Minimum in Rotated Sorted Array
- Search in Rotated Sorted Array
- Search in Rotated Sorted Array II
- Peak Index in a Mountain Array
- Find Peak Element
- Random Pick with Weight
- Time Based Key-Value Store
- Search a 2D Matrix
- Search a 2D Matrix II
- Find K Closest Elements
- Kth Smallest Element in a Sorted Matrix
- Count of Range Sum
- Median of Two Sorted Arrays
DFS
- Guidelines
- Invert A Binary Tree
- Symmetric Tree
- Same Tree
- Subtree of Another Tree
- Merge Two Binary Trees
- Maximum Depth of Binary Tree
- Balanced Binary Tree
- Path Sum
- Convert Sorted Array to Binary Search Tree
- Diameter of Binary Tree
- Flood Fill
- Lowest Common Ancestor of a Binary Tree
- Validate Binary Search Tree
- Lowest Common Ancestor of a Binary Search Tree
- Maximum Binary Tree
- Path Sum II
- Path Sum III
- Construct Binary Tree from Preorder and Inorder Traversal
- All Nodes Distance K in Binary Tree
- Kth Smallest Element in a BST
- Binary Tree Maximum Path Sum
- Serialize and Deserialize Binary Tree
- Longest Increasing Path in a Matrix
Dynamic Programming
- Guidelines
- Climbing Stairs
- Counting Bits
- House Robber
- House Robber II
- Coin Change
- Decode Ways
- Maximum Subarray
- Maximum Product Subarray
- Combination Sum IV
- Best Time to Buy and Sell Stock with Cooldown
- Unique Paths
- Longest Increasing Subsequence
- Longest Palindromic Substring
- Palindromic Substrings
- Maximal Square
- Target Sum
- Partition Equal Subset Sum
- Word Break
- Number of Longest Increasing Subsequence
- Partition to K Equal Sum Subsets
- Longest Valid Parentheses
- Maximum Profit in Job Scheduling
Graphs
- Guidelines
- Pacific Atlantic Water Flow
- Number of Islands
- Clone Graph
- Course Schedule
- Course Schedule II
- Cheapest Flights Within K Stops
- Minimum Height Trees
- Sort Items by Groups Respecting Dependencies
- Bus Routes
Greedy
- Guidelines
- Best Time To Buy And Sell Stock
- Gas Station
- Jump Game
- Largest Number
- Minimum Number of Arrows to Burst Balloons
- Task Scheduler
- Course Schedule III
Heaps
- Guidelines
- K Closest Points to Origin
- Find K Pairs with Smallest Sums
- Top K Frequent Elements
- Kth Largest Element in an Array
- Sort Characters By Frequency
- Reorganize String
- Merge k Sorted Lists
- Maximum Frequency Stack
- Find Median from Data Stream
- Sliding Window Median
- Smallest Range Covering Elements from K Lists
Intervals
- Guidelines
- Insert Interval
- Interval List Intersections
- Merge Intervals
- Non-overlapping Intervals
Linked List
- Guidelines
- Reverse Linked List
- Middle of the Linked List
- Palindrome Linked List
- Linked List Cycle
- Merge Two Sorted Lists
- Remove Linked List Elements
- Remove Duplicates from Sorted List
- Add Two Numbers
- Odd Even Linked List
- Remove Nth Node From End of List
- Rotate List
- Swap Nodes in Pairs
- Sort List
- Reorder List
- Linked List Cycle II
- Reverse Linked List II
- LRU Cache
- Reverse Nodes in k-Group
Math
- Guidelines
- Reverse Integer
- Pow(x, n)
- Insert Delete GetRandom O(1)
- Basic Calculator II
- Basic Calculator
Sliding Window
- Guidelines
- Permutation in String
- Minimum Size Subarray Sum
- Fruit Into Baskets
- Longest Repeating Character Replacement
- Longest Substring Without Repeating Characters
- Find All Anagrams in a String
- Minimum Window Substring
- Sliding Window Maximum
- Count Unique Characters of All Substrings of a Given String
- Minimum Number of K Consecutive Bit Flips
- Substring with Concatenation of All Words
Trie
- Guidelines
- Implement Trie (Prefix Tree)
- Design Add and Search Words Data Structure
- Longest Word in Dictionary
- Maximum XOR of Two Numbers in an Array
- Concatenated Words
- Word Search II
- Prefix and Suffix Search
- Palindrome Pairs
Two Pointers
- Guidelines
- Valid Palindrome
- Squares of a Sorted Array
- Backspace String Compare
- 3Sum
- 3Sum Closest
- Container With Most Water
- Next Permutation
- Subarray Product Less Than K
- Sort Colors
- Trapping Rain Water